4. Warping and EstimationΒΆ
Carefully read the lecture notes on Geometrical Operators and on Homogeneous Coordinates.
Exercise Affine Transform
Write a function affineTransform(f, x1, y1, x2, y2, x3, y3,
width, height)
that warps the parallelogram defined by the three
points (x1,y1), (x2,y2) and (x3,y3) onto a new image of given width
and height. Here we assume that the coordinates are given with
respect to the graphical axis (x from left to right, y from top to
bottom).
The mapping of the points \((x_i, y_i)\) in the image \(f\) to the points \((x'_i,y'_i)\) in the resultant image should be:
i | \((x_i,y_i)\) | \((x'_i,y'_i)\) |
---|---|---|
1 | \((x_1,y_1)\) | \((0,0)\) |
2 | \((x_2,y_2)\) | \((width-1,0)\) |
3 | \((x_3,y_3)\) | \((0,height-1)\) |
Calculation of the parameters of the affine transform should be done by your own code (do not use the code from OpenCV or other sources).
Be sure that your code works with:
- image of unequal width and height
- scalar and color images
- situations where \((x_i,y_i)\) are chosen outside the original image (e.g. when you want to rotate the image around its center).
In your report present the code and the formula’s on which it is based together with some examples showing that it works as intented.
You don’t have to write your own warping algorithm. Only the calculation of the parameters has the be done. It is up to you to use a warp function from scikit-image or OpenCV. If you do write your own warping function be sure to include at least bilinear interpolation.
Exercise Perspective Transform
Write a function perspectiveTransform(f, x1, y1, x2, y2, x3, y3,
x4, y4, width, height)
that warps a quadrilateral with vertices
at (x1,y1), (x2,y2), (x3,y3) and (x4,y4) to a new image of given
width and height. The mapping is given by the following table:
i | \((x_i,y_i)\) | \((x'_i,y'_i)\) |
---|---|---|
1 | \((x_1,y_1)\) | \((0,0)\) |
2 | \((x_2,y_2)\) | \((width-1,0)\) |
3 | \((x_3,y_3)\) | \((0,height-1)\) |
4 | \((x_4,y_4)\) | \((width-1, height-1)\) |
The same coordinate system is used as in the previous exercise.
Calculation of the parameters of the perspective transform should be done by your own code (do not use the code from OpenCV or other sources).
Be sure that your code works with:
- image of unequal width and height
- scalar and color images
In your report present the code and the formula’s on which it is based together with some examples showing that it works as intented. You should at least be able to redo the following example (make a reasonable choice for width and heigth of the new image assuming the flyer is of A4 shape).
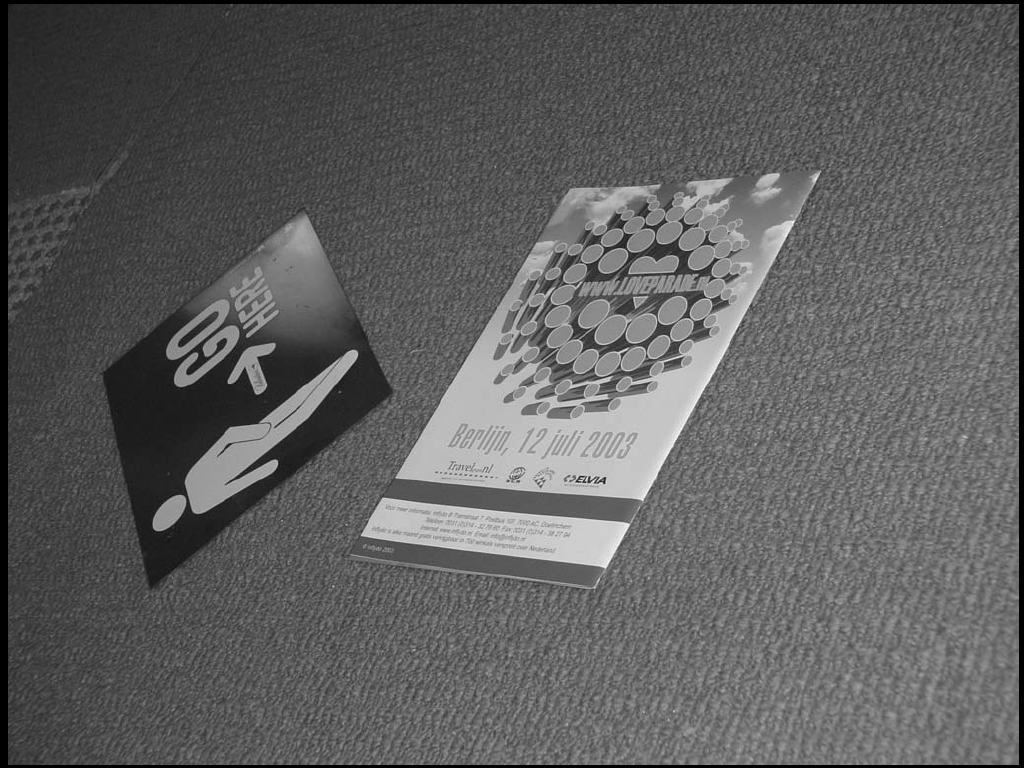
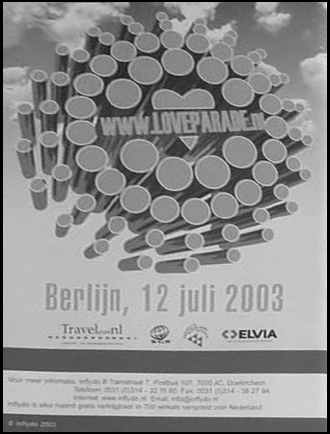
You don’t have to write your own warping algorithm. Only the calculation of the parameters has the be done. It is up to you to use a warp function from scikit-image or OpenCV. If you do write your own warping function be sure to include at least bilinear interpolation.