5.5.3. Canonical Systems¶
In classical control theory two systems are the canonical systems: the first and second order systems (higher order systems can be constructed by cascading these canonical systems).
5.5.3.1. First Order Systems¶
A first order system is characterized with a differential equation having only first order derivatives. Although simple these first order systems are of great importance. E.g. a mass-damper system and a mass heating system are examples of first order systems. And often higher order systems can be approximated with a first order system.
The canonical first order system is characterized with transfer function:
where \(\tau\) is the time constant of the system and \(K\) is the DC gain of the system.
The impulse response can be derived using the Laplace transform pair
We can rewrite \(H(s)\) as
and thus the impulse response of a first order system is:
To calculate the step response of the first order system we use the fact that the Laplace transform of the unit step equals \(1/s\). And thus the Laplace transform of the step response is
This Laplace transform is not in our table of common transform pairs. But if we calculate the partial fractions of this we are lookking for factors \(A\) and \(B\) such that
meaning that
leading to \(A=1\) and \(B=-\tau\). So the Laplace transform of the step response equals:
Now we have two terms that are in the table of Laplace transform pairs and the step response of a first order system is:
Show code for figure
1import numpy as np
2import matplotlib.pyplot as plt
3from scipy import signal
4import control
5
6plt.clf()
7
8tau = 1
9H = control.tf([1], [tau, 1])
10t, ystep = control.step_response(H)
11plt.plot(t, ystep, label=r'$\tau=1$');
12
13tau=2
14H = control.tf([1], [tau, 1])
15t, ystep = control.step_response(H)
16plt.plot(t, ystep, label=r'$\tau=2$');
17plt.legend();
18plt.savefig('source/figures/firstorder_stepresponse.png')
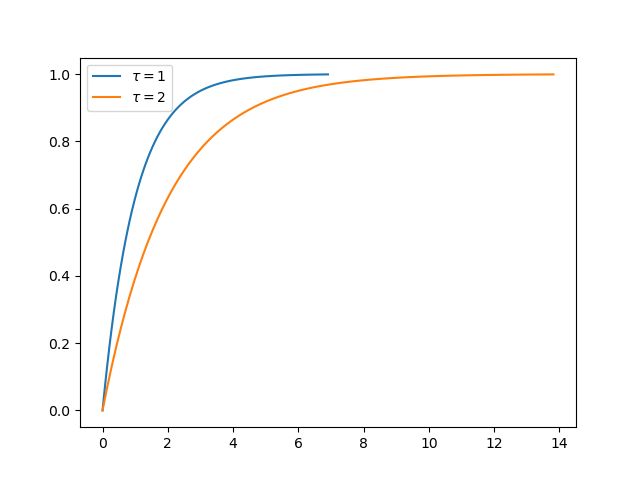
Fig. 5.51 Step Response of First Order System.¶
5.5.3.2. Second Order Systems¶
A canonical second order system is characterized with transfer function
where \(\w_0\) is the undamped (natural) frequency and \(\beta\) is the damping parameter.
This system has two poles that determine the behaviour of the system. Here study its impulse response and step response.
The poles can be calculated with the quadratic formula:
We can distinguish four different situations depending on the value of \(\beta\).
- \(\beta=0\) Undamped
For an undamped system we get:
\[H(s) = \frac{\w_0^2}{s^2+\w_0^2}\]such that the Laplace transform of the step response equals
\[\frac{H(s)}{s} = \frac{\w_0^2}{s(s^2+\w_0^2)}\]We want to write this like
\[\frac{A}{s} + \frac{Bs}{s^2+\w_0^2}\]leading to
\[As^2 + A\w_0^2 + Bs^2 = \w_0^2\]such that
\[A=1, \quad B=-1\]The Laplace transform of the step response then becomes:
\[\frac{H(s)}{s} = \frac{1}{s} - \frac{s}{s^2+\w_0^2}\]From the table of Laplace transform pairs we find the step response of an undamped second order system:
\[\left( 1 - \cos(\w_0 t) \right) u(t)\]Evidently this is where the term undamped comes from: a step at the input leads to a sinusoidal response with infinite duration.
Show code for figure
1import numpy as np 2import matplotlib.pyplot as plt 3from scipy import signal 4import control 5 6plt.clf() 7 8w0 = np.pi 9b = 0 10t = np.linspace(0, 20, 1000) 11H = control.tf([w0**2], [1, 2*b*w0, w0**2]) 12_, ystep = control.step_response(H, t) 13plt.plot(t, ystep, label=r'$\omega_0=\pi$'); 14 15w0 = np.pi/2 16H = control.tf([w0**2], [1, 2*b*w0, w0**2]) 17_, ystep = control.step_response(H, t) 18plt.plot(t, ystep, label=r'$\omega_0=\pi/2$'); 19plt.legend(); 20 21plt.savefig('source/figures/secondorder_undamped.png')
Fig. 5.52 Step Response of Second Order Undamped System.¶
- \(0<\beta<1\) Underdamped
For \(\beta\) in between zero and one the system is underdamped. It will oscillate but the amplitude will decrease over time.
Show code for figure
1import numpy as np 2import matplotlib.pyplot as plt 3from scipy import signal 4import control 5 6plt.clf() 7 8w0 = np.pi 9t = np.linspace(0, 10, 1000) 10 11for b in [0.20, 0.50]: 12 H = control.tf([w0**2], [1, 2*b*w0, w0**2]) 13 _, ystep = control.step_response(H, t) 14 plt.plot(t, ystep, label=r'$\beta={}$'.format(b)); 15 16plt.legend(); 17 18plt.savefig('source/figures/secondorder_underdamped.png')
Fig. 5.53 Step Response of Second Order Underdamped System.¶
- \(\beta = 1\) Critically Damped
In case \(\beta=1\) there are two identical real valued poles:
\[p_{1,2} = -\w_0\]and we can write
\[H(s) = \frac{\w_0^2}{(s+\w_0)(s+\w_0)} = \frac{\w_0}{s+\w_0}\frac{\w_0}{s+\w_0} = \frac{1}{1+s/\w_0} \frac{1}{1+s/\w_0}\]showing that a critically damped second order system is the cascade of two identical first order system each with time constant \(1/\w_0\).
Show code for figure
1import numpy as np 2import matplotlib.pyplot as plt 3from scipy import signal 4import control 5 6plt.clf() 7w0 = np.pi 8b = 1 9t = np.linspace(0, 5, 1000) 10 11H = control.tf([w0**2], [1, 2*b*w0, w0**2]) 12_, ystep = control.step_response(H, t) 13plt.plot(t, ystep, label=r'$\omega=\pi$'); 14 15w0 = np.pi/2 16H = control.tf([w0**2], [1, 2*b*w0, w0**2]) 17_, ystep = control.step_response(H, t) 18plt.plot(t, ystep, label=r'$\omega=\pi/2$'); 19plt.legend(); 20 21plt.savefig('source/figures/criticallydamped2ndstep.png')
Fig. 5.54 Step Response of Second Order Critically Damped System.¶
- \(\beta>1\) Overdamped
For \(\beta>1\) we have two poles both of which are real valued:
\[p_{1,2} = \w_0\left(-\beta \pm \sqrt{\beta^2-1} \right)\]Show code for figure
1import numpy as np 2import matplotlib.pyplot as plt 3from scipy import signal 4import control 5 6plt.clf() 7 8w0 = np.pi 9 10b = 1 11t = np.linspace(0, 10, 1000) 12 13H = control.tf([w0**2], [1, 2*b*w0, w0**2]) 14_, ystep = control.step_response(H, t) 15plt.plot(t, ystep, '--', label=r'$\beta={}$ (critically damped)'.format(b)); 16 17b = 2 18t = np.linspace(0, 10, 1000) 19 20H = control.tf([w0**2], [1, 2*b*w0, w0**2]) 21_, ystep = control.step_response(H, t) 22plt.plot(t, ystep, label=r'$\beta={}$'.format(b)); 23 24b = 4 25H = control.tf([w0**2], [1, 2*b*w0, w0**2]) 26_, ystep = control.step_response(H, t) 27plt.plot(t, ystep, label=r'$\beta={}$'.format(b)); 28plt.legend(); 29 30 31plt.savefig('source/figures/overdamped2ndstep.png')
Fig. 5.55 Step Response of Second Order Overdamped System. The critically damped response is shown for comparison.¶